Making a Scrollable Container in HTML
Table of Contents
- Introduction
- Understanding Scrollable Containers
- Why Use Scrollable Containers?
- Basic HTML Structure for Scrollable Containers
- Styling Scrollable Containers with CSS
- Advanced Scrollable Container Techniques
- Common Use Cases of Scrollable Containers
- Accessibility Considerations
- Optimizing Performance for Scrollable Containers
- Tools and Libraries to Enhance Scrollable Containers
- Step-by-Step Tutorial: Creating a Scrollable Container
- Troubleshooting Common Issues
- Best Practices for Scrollable Containers
- Conclusion
- Call to Action
Introduction
Ever been on a website and thought, "Wow, that scrolls so nice!"? Or maybe you've struggled to fit too much stuff into a small space on your page. Well, that's where scrollable containers come in handy!
These days, making websites look good and work well is super important. A good scrollable container can make your site look better and be easier to use. Whether you're showing off products, sharing photos, or just dealing with lots of text, scrollable containers are your best friend.
But hold on a sec—before we jump in, let's take a step back. What exactly are these scrollable containers, and why do we need them in modern web design? Grab a drink, and let's dive into this scrolling adventure together!
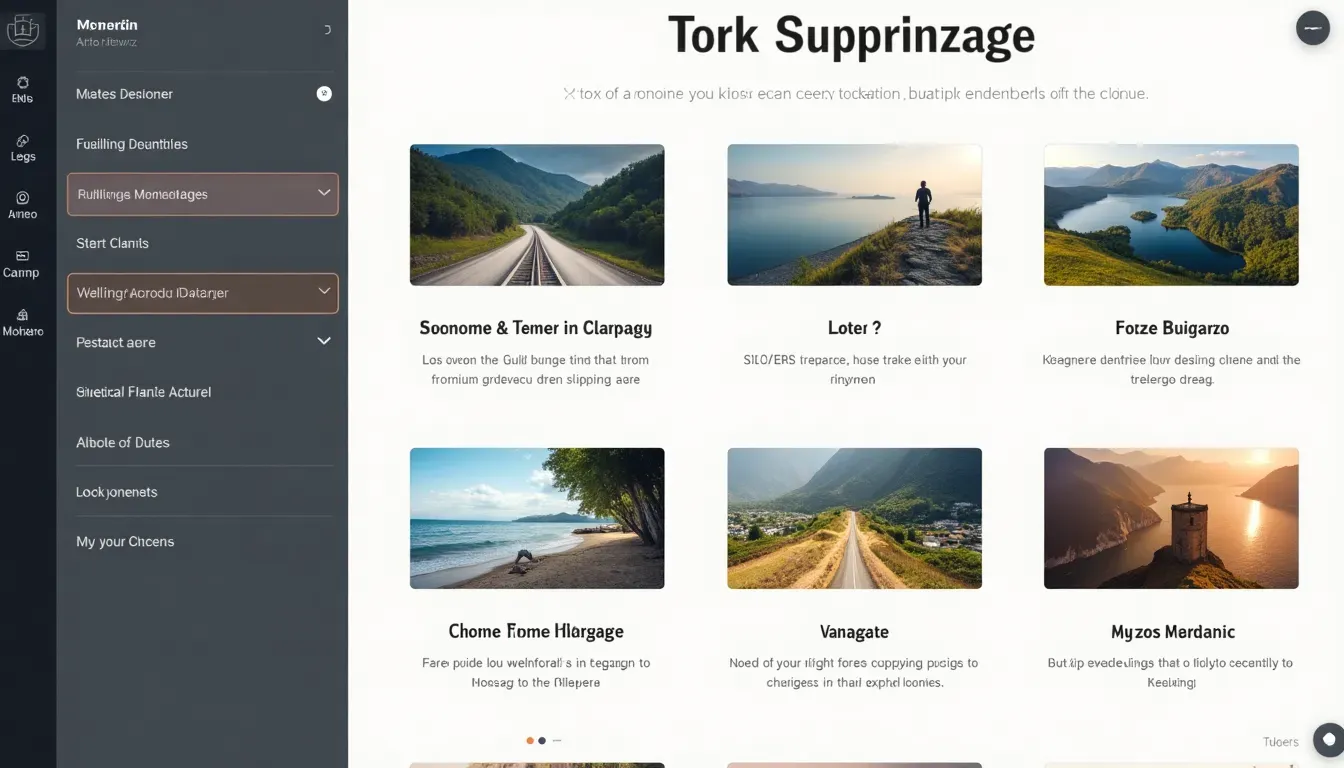
Understanding Scrollable Containers
At its heart, a scrollable container is just a part of your webpage that lets content overflow and be scrolled through. Think of it like a window that users can look through to see more stuff without messing up the rest of the page.
Imagine your favorite bookstore. The shelves are packed with books, but there's only so much space. Instead of cramming everything in, they use those cool rotating displays—letting you browse through tons of books without feeling overwhelmed. Scrollable containers do the same thing for your website.
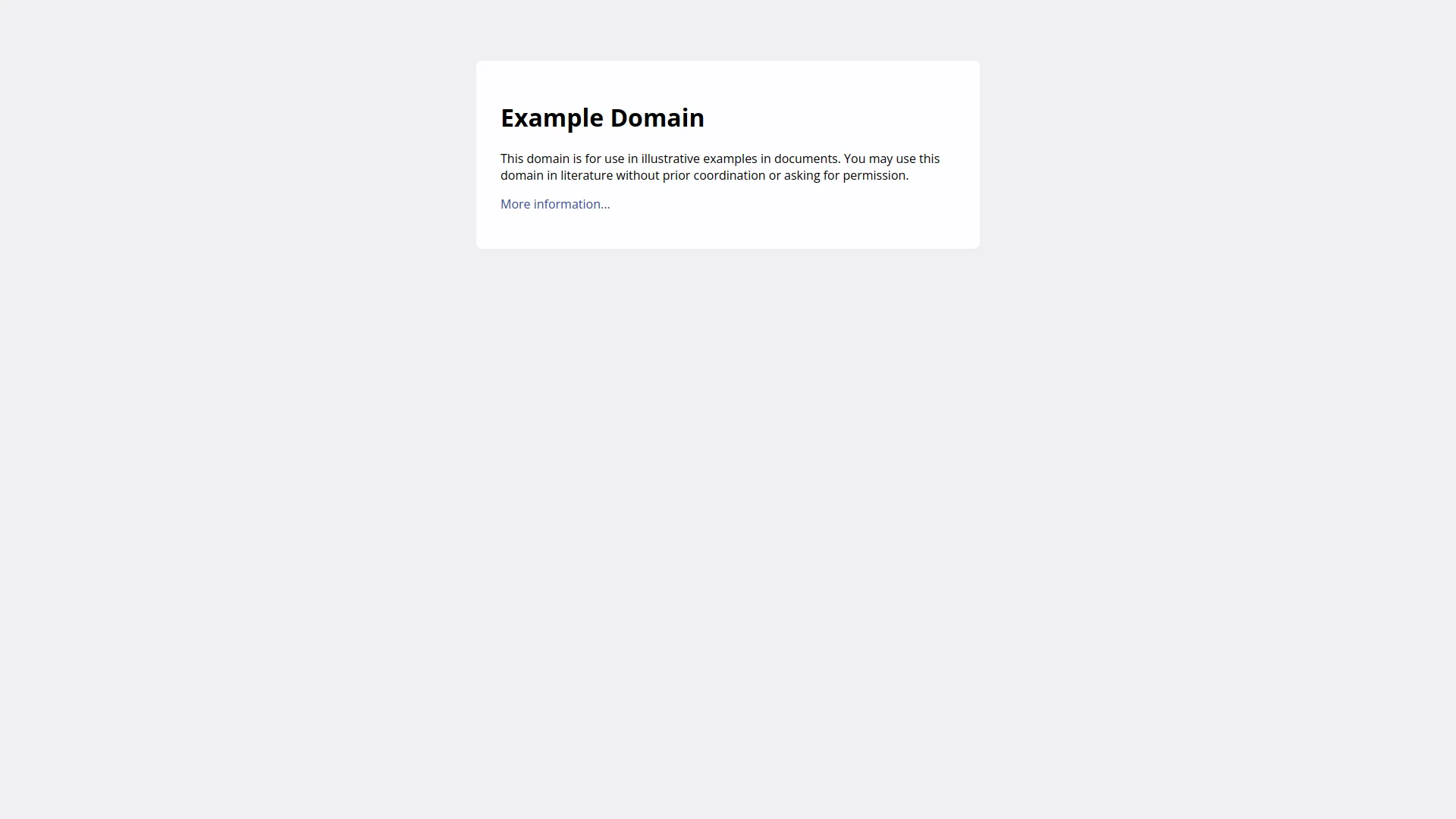
Key stuff to remember:
- Overflow Management: Controls how extra content is handled.
- User Navigation: Lets users easily access hidden or extra content.
- Design Flexibility: Gives designers a way to create organized and good-looking layouts.
Why Use Scrollable Containers?
You might be thinking, "Why not just let everything flow naturally?" Good question! Here's why scrollable containers are awesome:
1. Better User Experience
Scrollable containers can make your site way easier to use by organizing content into manageable chunks. Instead of one long, scary list, you break it up into scrollable bits, making it easier for people to find what they want.
2. Saves Space
Webpages only have so much room. Scrollable containers help you fit more stuff in without making the page look messy.
3. Looks Good
A well-made scrollable container can make your website look fancy. Smooth scrolling and custom scrollbars can make your site look better and match your overall design.
4. Faster Loading
Loading content in sections can make your site faster. Instead of loading everything at once (which can slow things down), scrollable containers can help load content as people need it.
Basic HTML Structure for Scrollable Containers
Before we get into the fancy stuff, let's start with the basics. Here's a simple example of how to set up a scrollable container:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Scrollable Container Example</title>
<style>
.scrollable-container {
width: 300px;
height: 200px;
border: 1px solid #ccc;
overflow: auto;
}
</style>
</head>
<body>
<div class="scrollable-container">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Suspendisse...</p>
<!-- Add more content here -->
</div>
</body>
</html>
What's going on here:
-
<div class="scrollable-container">
: This<div>
is our scrollable container. -
CSS Styling
:
-
width
&height
: Sets how big the container is. -
border
: Adds a visible edge so we can see the container. -
overflow: auto;
: This is the key part - it decides how extra content is handled.
-
With this setup, anything that's too big for the container will automatically become scrollable. Pretty simple, right? But wait—there's so much more you can do!
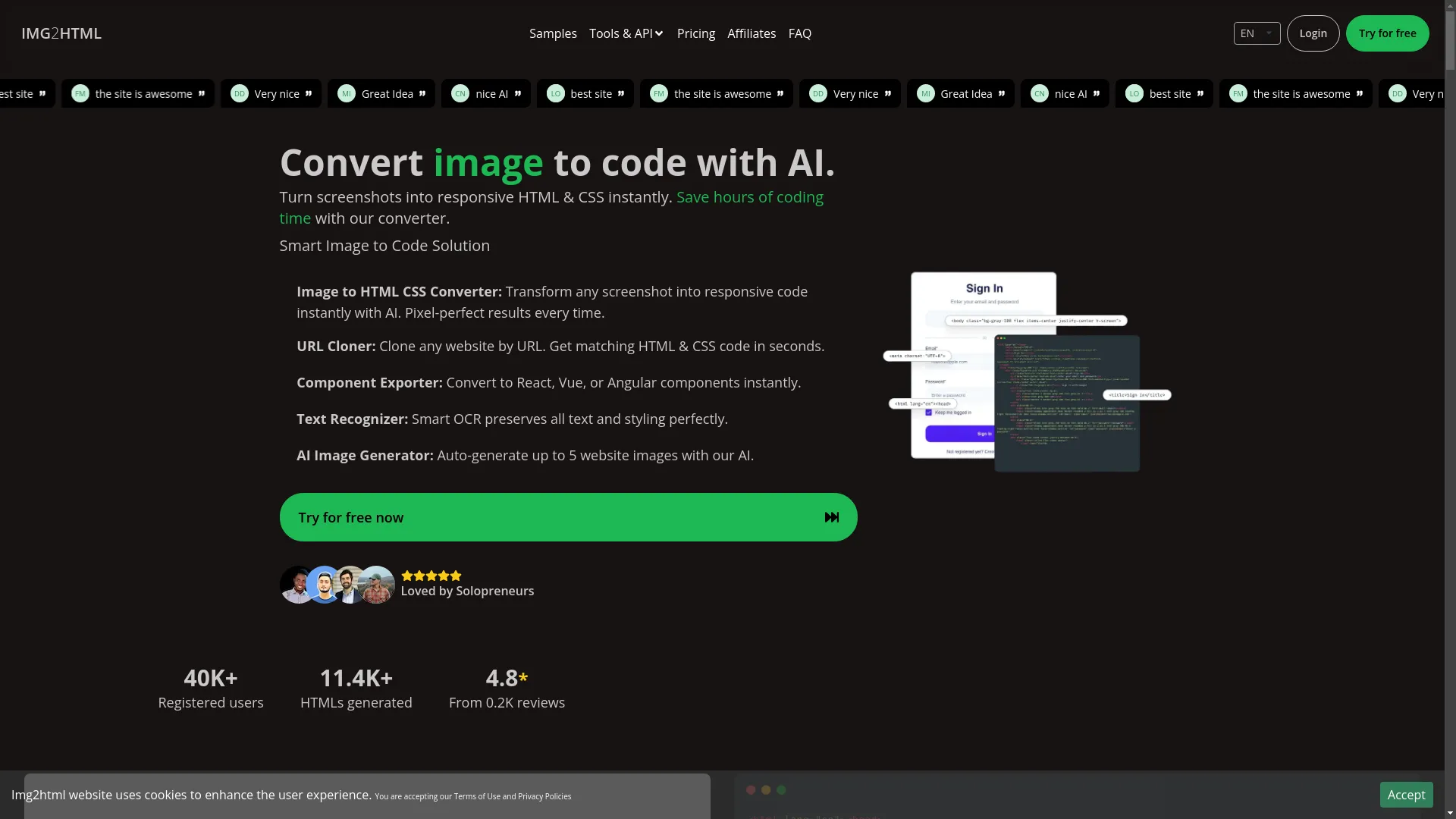
Styling Scrollable Containers with CSS
CSS is where the magic happens. By tweaking a few things, you can turn a plain scrollable container into something really cool and user-friendly. Let's look at the important CSS stuff that controls how scrolling works.
Overflow Property
The
overflow
property is super important for scrollable containers. It decides what happens to content that's too big for the container. Here's what you can do with it:
-
visible
: This is the default. Content might stick out of the container. -
hidden
: Cuts off extra content without showing a scrollbar. -
scroll
: Always shows scrollbars, even if you don't need them. -
auto
: Shows scrollbars only when you need them (when there's too much content).
Example:
.scrollable-container {
overflow: auto; /* Scrollbars only show up when needed */
}
Pro Tip:
Usually,
overflow: auto;
is your best bet for a clean look. It only shows scrollbars when you actually need them.
Setting Height and Width
You gotta set the size of your scrollable container. If you don't, the
overflow
property won't do anything.
.scrollable-container {
width: 400px;
height: 300px;
overflow: auto;
}
Responsive Design Tip:
While using exact sizes is easy, for responsive designs you might want to use percentages or viewport units (
vw
,
vh
) so things adjust to different screen sizes.
Scrollbar Styling
Making the scrollbar look good can make your whole container look better. You can't do too much with scrollbars across all browsers, but here's how you can start, especially for browsers like Chrome and Safari:
.scrollable-container::-webkit-scrollbar {
width: 12px;
}
.scrollable-container::-webkit-scrollbar-track {
background: #f1f1f1;
}
.scrollable-container::-webkit-scrollbar-thumb {
background: #888;
border-radius: 6px;
}
.scrollable-container::-webkit-scrollbar-thumb:hover {
background: #555;
}
Note:
This scrollbar styling stuff (using
::-webkit-scrollbar
) doesn't work on all browsers. For a more consistent look, you might want to use special scrollbar libraries.
Advanced Scrollable Container Techniques
Once you've got the basics down, let's look at some cooler stuff you can do to make your scrollable containers even better.
Smooth Scrolling
Smooth scrolling makes things feel nicer when users scroll through content. It makes navigation feel more natural and less jumpy.
How to do it:
.scrollable-container {
scrollbar-width: thin;
scrollbar-color: #888 #f1f1f1;
scroll-behavior: smooth;
}
Browser Support:
Most modern browsers support
scroll-behavior
, but it's always good to check, especially for older browsers.
Custom Scrollbars
If you want total control over how scrollbars look, you can use libraries like SimpleBar or OverlayScrollbars . These let you style scrollbars the same way across different browsers and add extra features.
Example using SimpleBar:
-
Add SimpleBar to your page:
<link rel="stylesheet" href="https://unpkg.com/simplebar@latest/dist/simplebar.css" /> <script src="https://unpkg.com/simplebar@latest/dist/simplebar.min.js"></script>
-
Use it on your container:
<div class="scrollable-container" data-simplebar> </div>
Why it's cool:
- Works the same on different browsers.
- Lots of ways to customize it.
- Easy to use and doesn't slow down your site.
Responsive Scrollable Containers
With so many different devices out there, making sure your scrollable containers work well on all screen sizes is super important. Using relative sizes and media queries can help with this.
Example:
.scrollable-container {
width: 90%;
max-width: 800px;
height: 50vh;
overflow: auto;
}
@media (max-width: 600px) {
.scrollable-container {
height: 60vh;
}
}
Key Points:
- Relative Units: Use percentages or viewport units instead of exact pixels so containers can adjust to screen size.
- Media Queries: Change the container's size and behavior for specific screen sizes to make sure it looks good on all devices.
Common Use Cases of Scrollable Containers
Scrollable containers are super useful in web design. Let's look at some common ways people use them.
Navigation Menus
Ever tried to use a website with a ton of menu items? Scrollable navigation menus can keep things organized without overwhelming users.
<nav class="scrollable-menu">
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<!-- More menu items -->
</ul>
</nav>
Styling Tip:
Set a max height for the menu and use
overflow-y: auto;
to let it scroll up and down when needed.
Image Galleries
Showing lots of images can be tricky. Scrollable containers let users browse through images side-to-side or up-and-down without cluttering the page.
<div class="scrollable-gallery">
<img src="image1.jpg" alt="Image 1">
<img src="image2.jpg" alt="Image 2">
<!-- More images -->
</div>
CSS Snippet:
.scrollable-gallery {
display: flex;
gap: 10px;
overflow-x: auto;
white-space: nowrap;
}
.scrollable-gallery img {
height: 150px;
flex-shrink: 0;
}
UX Tip: Use lazy loading for images to make things faster, especially if you have lots of big images.
Content Sections
Breaking up long content into scrollable sections can make it easier to read and more engaging. It lets users focus on specific parts without feeling overwhelmed.
<section class="scrollable-content">
<h2>Chapter 1</h2>
<p>...</p>
<!-- More chapters -->
</section>
Design Tip: Use padding and background colors to make different content sections stand out and easy to navigate.
Accessibility Considerations
Making scrollable containers isn't just about making them look good and work well; it's also about making sure everyone can use your website, including people with disabilities.
Keyboard Navigation
Make sure people can move through scrollable containers using keyboard controls. For example, make sure pressing arrow keys scrolls the content.
Implementation Tip:
.scrollable-container:focus {
outline: 2px solid #000;
}
Screen Reader Compatibility
Label scrollable containers properly so screen readers can understand them.
<div class="scrollable-container" aria-label="Scrollable Content">
<!-- Content -->
</div>
Enough Contrast: Make sure text in scrollable containers is easy to read against the background, especially if you're changing scrollbar colors.
Use tools like WebAIM's Contrast Checker to make sure color combinations work well.
Optimizing Performance for Scrollable Containers
Making sure scrollable containers work smoothly is super important, especially when they have lots of content. Here are some ways to keep things fast and efficient.
Lazy Loading Content
Instead of loading everything at once, load content as people scroll. This makes initial load times faster and uses less data.
How to do it with Intersection Observer:
const lazyContainers = document.querySelectorAll('.scrollable-container');
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
// Load content
entry.target.classList.add('loaded');
observer.unobserve(entry.target);
}
});
});
lazyContainers.forEach(container => {
observer.observe(container);
});
Minimize Reflows and Repaints
Changing the DOM too much can slow down your site. Try to optimize your JavaScript and CSS to avoid unnecessary changes in scrollable containers.
Use Hardware Acceleration
Use GPU acceleration for smoother scrolling, especially for animations or transitions in scrollable containers.
Example:
.scrollable-container {
transform: translate3d(0, 0, 0);
}
Tools and Libraries to Enhance Scrollable Containers
There are some cool tools and libraries out there that can make working with scrollable containers easier. Here are some popular ones:
SimpleBar
A lightweight library that gives you custom scrollbars without changing how scrolling normally works.
- Small and fast.
- Easy to use.
- Works the same on different browsers.
OverlayScrollbars
A versatile library that lets you customize scrollbars in lots of ways.
- Works with touch screens.
- Supports right-to-left languages.
- Lots of customization options.
Perfect Scrollbar
Designed to feel natural, Perfect Scrollbar makes custom scrollbars that fit in with your site's design.
- Doesn't change your HTML.
- Easy to add to your site.
- Looks and feels consistent.
Pro Tip: Always pick libraries that are kept up-to-date and have good community support to make sure they're compatible and secure.
Step-by-Step Tutorial: Creating a Scrollable Container
Ready to get your hands dirty and make a scrollable container from scratch? Let's walk through a practical example using HTML and CSS.
What We're Making
We're going to create a stylish, responsive scrollable container that shows a list of articles.
Step 1: HTML Structure
First, let's set up the basic HTML structure.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Scrollable Container Tutorial</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="scrollable-container">
<article>
<h2>Article 1</h2>
<p>Content for article 1...</p>
</article>
<article>
<h2>Article 2</h2>
<p>Content for article 2...</p>
</article>
<!-- Add more articles as needed -->
</div>
</body>
</html>
Step 2: CSS Styling
Now, let's style the container to make it scrollable and look good.
/* styles.css */
body {
font-family: Arial, sans-serif;
background-color: #f9f9f9;
display: flex;
justify-content: center;
padding: 50px;
}
.scrollable-container {
width: 80%;
max-width: 800px;
height: 400px;
border: 2px solid #ddd;
border-radius: 8px;
background-color: #fff;
overflow-y: auto;
padding: 20px;
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
}
.scrollable-container article {
margin-bottom: 20px;
}
.scrollable-container h2 {
margin-bottom: 10px;
color: #333;
}
.scrollable-container p {
color: #555;
line-height: 1.6;
}
What's happening here:
- Container Size: The container is centered and has a fixed height to make it scroll vertically.
- Making it Look Good: Border, rounded corners, padding, and shadow make it look nice.
- Content Styling: Margins and colors make it easy to read and organized.
Step 3: Test Your Scrollable Container
Add enough articles to make scrolling necessary. Open your HTML file in a browser to see your scrollable container in action.
What You Should See:
You should have a nice-looking container with multiple articles. As you add more articles, a scrollbar should appear, letting users scroll through the content smoothly.
Make it Better: To make the container work well on different screen sizes, adjust the width and height using percentages and media queries as needed.
You can try this feature yourself at Img2html to quickly convert your designs into responsive HTML and CSS code.
Troubleshooting Common Issues
Sometimes things don't work quite right when making scrollable containers. Here are some common problems and how to fix them.
1. Scrollbars Don't Show Up
Why it happens: The content inside isn't bigger than the container.
How to fix it:
- Make sure the container has a set height or width to allow overflow.
- Add more content to make scrolling necessary.
2. Scrollbars Mess Up the Layout
Why it happens: Scrollbars might take up space, causing things to shift around.
How to fix it:
-
Use
box-sizing: border-box;
to include padding and border in the element's total size. - Try using overlay scrollbars with custom libraries to avoid layout issues.
3. Scrollable Container Doesn't Work on Different Screen Sizes
Why it happens: Fixed sizes don't adjust to different screens.
How to fix it:
- Use percentages or viewport units instead of exact pixels.
- Use media queries to change styles based on screen size.
4. Scrollbars Look Different on Different Browsers
Why it happens: Different browsers show scrollbars differently, and some CSS might not work on all browsers.
How to fix it:
- Use libraries that work across browsers for custom scrollbars.
- Have backup styles for browsers that don't support certain scrollbar customizations.
5. Slow Performance with Lots of Content
Why it happens: Too much heavy content in scrollable containers can make scrolling slow.
How to fix it:
- Use lazy loading for images and other media.
- Optimize your content by minimizing heavy scripts and styles.
Best Practices for Scrollable Containers
To make sure your scrollable containers work well and are user-friendly, follow these best practices:
- Don't Overdo It - Avoid having too many scrollable areas inside each other. It can confuse users. Try to stick to one main scrollable area to keep things clear.
- Give Visual Hints - Use subtle clues like fading edges or partially cut-off content to show that there's more to see by scrolling.
- Make It Keyboard-Friendly - Make sure people can navigate through scrollable containers using just their keyboard. This helps users who rely on keyboards to get around.
- Make It Work Well on Touch Devices - Ensure that scrollable containers respond smoothly to touch gestures on phones and tablets.
- Use Clear Scroll Indicators - Customize scrollbars or add buttons (like "Load More") to make it obvious that there's more content to scroll through.
- Keep the Style Consistent - Make sure the design of scrollable containers matches the rest of your website to give a seamless experience.
- Test on Different Devices and Browsers - Regularly check how your scrollable containers work on various devices and browsers to ensure they're compatible and perform well.
Accessibility Considerations
Making your website accessible to everyone is super important. When designing scrollable containers, keep these things in mind:
1. Work with Screen Readers
Make sure screen readers can understand the content in scrollable containers. Use ARIA labels and roles where needed.
<div class="scrollable-container" role="region" aria-label="Article List">
<!-- Content -->
</div>
2. Keyboard Navigation
Users should be able to move through scrollable containers using keyboard shortcuts. Make sure focus states work and tab order makes sense.
3. Focusable Elements
All interactive elements inside scrollable containers should be focusable and accessible via keyboard.
4. Avoid Auto-Scrolling
Automatically scrolling content can be confusing, especially for people using assistive technologies. Let users control scrolling themselves.
5. Provide Skip Links
For keyboard users, provide skip links to let them bypass repetitive scrollable content if they want.
Conclusion
Creating scrollable containers in HTML is a key skill that can really improve your web design, making your content more organized, accessible, and good-looking. Whether you're a pro developer or just starting out, knowing how to make and style scrollable containers is super valuable.
From handling overflowing content to making user experience better with smooth and responsive scrolling, there's so much you can do. By following best practices and focusing on accessibility, you make sure your scrollable containers not only look good but also work well for everyone.
Remember, the trick is to balance how it works with how it looks, making sure your scrollable containers make the user experience better, not worse. So go ahead, try out different styles and techniques, and watch your web designs come to life with smooth scrolling!
Call to Action
Ready to take your web design skills up a notch? Start using scrollable containers in your projects today and see how they can make your sites more engaging and better looking. Whether you're showing off your work, writing a blog, or building a complex web app, scrollable containers are great for creating organized and dynamic layouts.
Don't stop here! Try out advanced styling techniques, play around with custom scrollbars, and make sure your designs work for everyone. And if you're looking for tools to make development easier, check out resources like Img2html , which uses smart AI to turn images into responsive HTML and CSS code super fast. Save time, make your workflow better, and focus on what really matters—creating awesome web experiences.
Happy coding!